Basic Concepts of Python Programming (Beginners Guide)
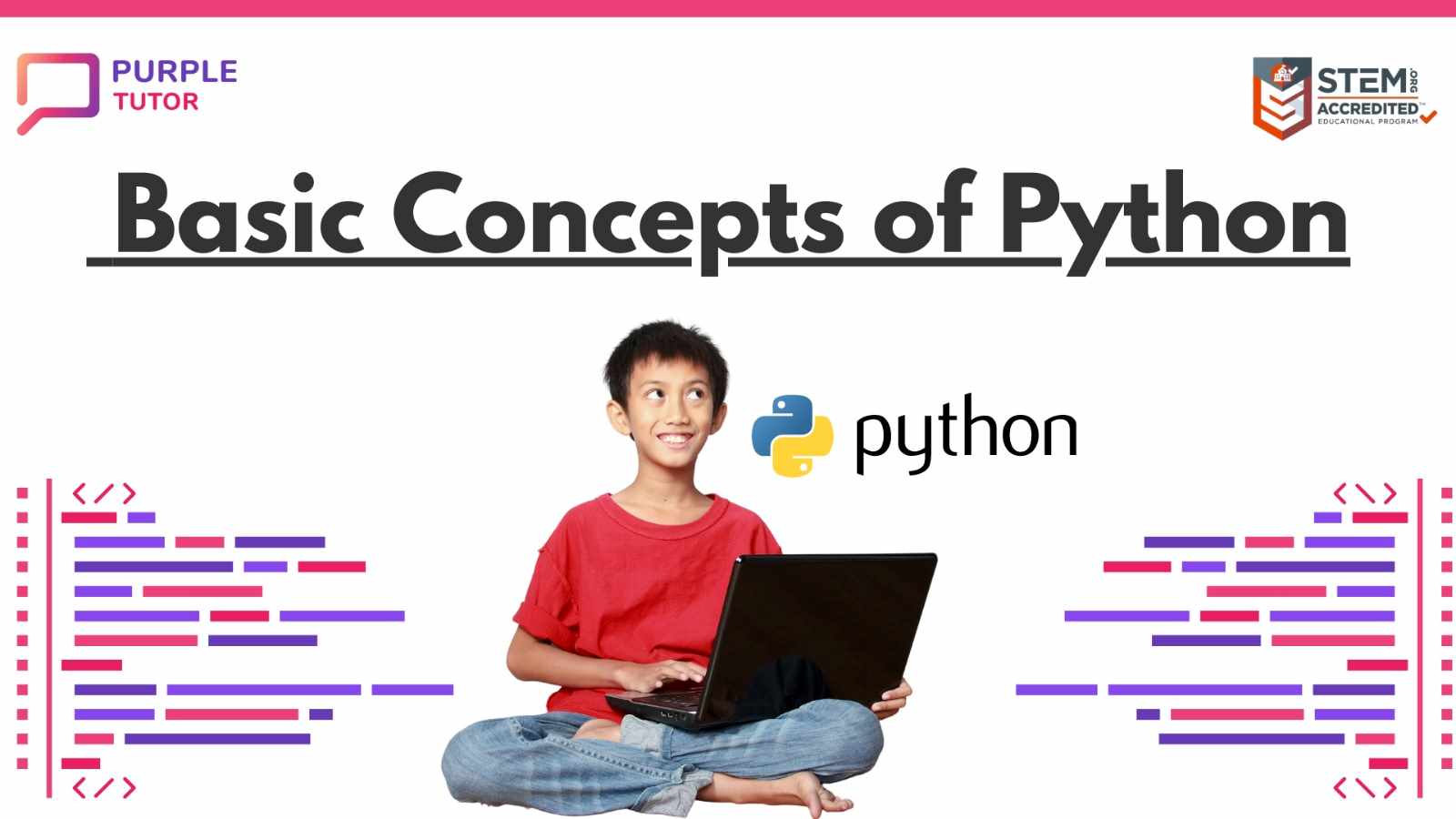
Python is a popular, high-level programming language known for its simplicity and readability. Python’s syntax is designed to be easy to understand and write, making it a great choice for beginners. It is also versatile and can be used for a wide variety of tasks, including web development, data analysis, machine learning, and scientific computing. This article will help you learn the basic concepts of Python.
Overview of Python Fundamentals
One of the key concepts in Python is the use of indentation to indicate blocks of code. Unlike other languages like C or Java, Python does not use curly braces or other symbols to define code blocks. Instead, it uses indentation to indicate the scope of a block of code. This makes Python code easy to read and understand, but it also means that it is important to be consistent with indentation.
Another important concept in Python is the use of variables. Variables are used to store data and can be used to reference that data throughout the program. Python uses a simple syntax for declaring variables, with no need for a specific keyword like “var” or “int”.
Python also has a wide variety of data types, including numbers (integers and floats), strings, lists, and dictionaries. These data types have specific methods and properties that can be used to manipulate and work with the data.
Python also has a number of built-in functions and modules that can be used to perform common tasks. For example, the “print()” function can be used to output data to the console, while the “math” module provides a variety of mathematical functions. It also supports object-oriented programming, which is a programming paradigm that uses objects and classes to organise and structure code. Python also has strong support for handling errors and exceptions. The “try-except” block can be used to handle and respond to errors that may occur in the program.
Basic Concepts of Python for Beginners
Here are some basic concepts in Python for beginners –
-
Variable
In Python, a variable is a container for storing a value. You can create a variable by assigning a value to it using the “=” operator. The value can be of any data type, such as a string, integer, or floating-point number. Once a variable is assigned a value, it can be used throughout your program.
For example:
x = 5
y = “Hello”
z = 3.14
In this example, x is assigned the value of 5, y is assigned the value “Hello”, and z is assigned the value 3.14.It’s important to note that variable names in Python must start with a letter or an underscore, and can only contain letters, numbers and underscores. They are case-sensitive.
You can also change the value of a variable by reassigning it to a new value. For example:
x = 5
x = 10
In this example, x is first assigned the value of 5 and then reassigned the value of 10. -
Data Types
Python has several built-in data types, including strings, integers, and floating-point numbers. In Python, data types refer to the type of value a variable holds. Python has several built-in data types:
- Numbers: This includes integers (e.g. 1, 2, 3) and floating-point numbers (e.g. 3.14, 1.23)
- Strings: A string is a sequence of characters (e.g. “hello”, “world”). Strings can be enclosed in single or double quotes
- Lists: A list is a collection of items that are ordered and changeable. Lists are written with square brackets and items are separated by commas
- Tuples: A tuple is similar to a list, but it’s immutable (i.e. its items cannot be changed). Tuples are written with round brackets and items are separated by commas
- Dictionaries: A dictionary is a collection of key-value pairs. Dictionaries are written with curly braces and keys and values are separated by colons
- Boolean: A Boolean data type is either True or False
- None: None is a special constant used to represent the absence of a value or a null value
You can check the data type of a variable using the built-in type() function.
For example:
x = 5
print(type(x))
# Output: <class ‘int’>;
y = “Hello”
print(type(y))
# Output: <class ‘str’>;
z = [1, 2, 3]
print(type(z))
# Output: <class ‘list’>;
In this example, x is an integer, y is a string, and z is a list.
-
Operators
Python supports various operators for performing mathematical and logical operations, such as +, -, *, /, and %. In Python, operators are special symbols that perform specific operations on one or more operands (i.e. the variables or values being operated on). Here are some common operators in Python:
- Arithmetic operators:
- Comparison operators:
- Logical operators:
- Assignment operators:
- Membership operators:
- Identity operators:
These operators perform basic mathematical operations, such as addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
These operators compare two values and return a Boolean value (True or False) based on the comparison. Examples include equal to (==), not equal to (!=), greater than (>), less than (<), greater than or equal to (>=), and less than or equal to (<=).
These operators are used to combine multiple conditions. Examples include and (and), or (or), and not (not).
These operators are used to assign a value to a variable. Examples include =, +=, -=, *=, /=, and %=.
These operators are used to test whether a value is in a sequence (e.g. a list or string). Examples include in and not in.
These operators are used to compare the identity of two objects. is and is not are the identity operators in python.
For example:
x = 5
y = 10
# Using the + operator to add x and y
result = x + y
print(result) # Output: 15
# Using the < operator to check if x is less than y
result = x < y
print(result) # Output: True
# Using the *= operator to multiply x by 2
x *= 2
print(x) # Output: 10
In this example, the first print statement uses the + operator to add x and y, the second print statement uses the < operator to check if x is less than y, and the third print statement uses the *= operator to multiply x by 2. -
Conditional Statements
It is used to execute different codes depending on certain conditions. The most common type of conditional statement is the if-else statement. The basic syntax of an if-else statement is as follows:
if condition:
# code to be executed if the condition is true
else:
# code to be executed if the condition is falseThe ‘condition’ in the if statement is a Boolean expression that evaluates to either True or False. If the condition is True, the code in the if block will be executed, otherwise, the code in the else block will be executed.
For example:
x = 5
if x > 0:
print(“x is positive”)
else:
print(“x is not positive”)
In this example, the condition ‘x > 0’ evaluates to True, so the code in the if block is executed and the output will be “x is positive”.You can also use ‘elif’ statement which is short for “else if” it allows you to check multiple conditions in the same if-else block.
x = 5
if x > 0:
print(“x is positive”)
elif x == 0:
print(“x is zero”)
else:
print(“x is negative”)
In this example, the first condition ‘x > 0’ is True, so the code in the first if block is executed and the output will be “x is positive”.It’s important to note that the ‘condition’ in the if statement can be any expression that evaluates to a Boolean value. You can use comparison operators, logical operators, and even function calls that return a Boolean value.
-
Loops
Python has two types of loops: for loops and while loops. These allow you to repeatedly execute a block of code. In Python, loops are used to repeatedly execute a block of code. There are two types of loops: for loops and while loops.
- For loops: A for loop is used to iterate over a sequence of items (e.g. a list, string, or tuple) and execute a block of code for each item. The basic syntax of a for loop is as follows:for variable in sequence:
# code to be executed for each item in the sequenceFor example:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number)
In this example, the for loop will iterate over the list of numbers and print each number. - While loops: A while loop is used to repeatedly execute a block of code as long as a certain condition is true. The basic syntax of a while loop is as follows:while condition:
# code to be executed as long as the condition is trueFor example:
x = 0
while x < 5:
print(x)
x += 1
In this example, the while loop will execute as long as ‘x < 5’ is true. The loop will first print 0, then 1, then 2, and so on until x becomes 5. At that point, the condition ‘x < 5’ becomes false and the loop stops.It’s important to note that if the condition in the while loop never becomes false, the loop will run indefinitely, causing an infinite loop. To avoid this, make sure the condition will eventually be false.
You can also use ‘break’ and ‘continue’ statements inside the loop to control the flow of the loop, ‘break’ statement is used to exit a loop and ‘continue’ statement is used to skip the current iteration and move on to the next one.
- For loops: A for loop is used to iterate over a sequence of items (e.g. a list, string, or tuple) and execute a block of code for each item. The basic syntax of a for loop is as follows:for variable in sequence:
-
Functions
In Python, a function is a block of code that can be reused throughout your program. Functions are useful for breaking down a complex program into smaller, more manageable pieces.
The basic syntax of a function is as follows:
def function_name(parameters):
# code to be executedHere, function_name is the name of the function, and ‘parameters’ are the input values that are passed to the function. The code inside the function is executed when the function is called.
For example:
def greet(name):
print(“Hello, ” + name)
greet(“John”)
In this example, the function ‘greet’ takes one parameter ‘name’, and when called it prints the string “Hello, ” followed by the value of the ‘name’ parameter.
Functions can also return a value by using the ‘return’ statement. This allows you to pass data back to the calling code. For example:
def add(x, y):
return x + y
result = add(3, 4)
print(result)
In this example, the function ‘add’ takes two parameters ‘x and y’, and returns the result of adding them together. The function is called with the values 3 and 4, and the returned value is stored in the variable ‘result’, which is then printed.
Functions can also have default values for the parameters, these default values are used when the caller does not provide a value for that parameter.
For example:
def greet(name, greeting = “Hello”):
print(greeting + “, ” + name)
greet(“John”)
In this example, the function ‘greet’ has one mandatory parameter ‘name’ and one optional parameter ‘greeting’ with a default value of “Hello”. When the function is called without providing a value for the greeting parameter, it will use the default value of “Hello”.
-
Libraries
- NumPy: A library for working with numerical data in Python. It provides tools for working with arrays, matrices, and mathematical functions.
- Pandas: A library for working with data in Python. It provides tools for data manipulation, data analysis, and data visualization.
- Matplotlib: A library for creating visualizations in Python. It provides tools for creating charts, plots, and other types of graphics.
- Scikit-learn: A library for machine learning in Python. It provides tools for classification, regression, clustering, and more.
- TensorFlow: A library for building and training machine learning models in Python. It’s widely used for deep learning, computer vision, natural language processing, and more.
- OpenCV: A library for computer vision in Python. It provides tools for image and video processing, feature detection and extraction, and more.
-
Classes and Objects
In Python, a class is a blueprint for creating objects, and an object is an instance of a class. Classes provide a way to model real-world concepts and encapsulate data and behaviour in a single unit. Object-oriented programming (OOP) is a programming paradigm that uses classes and objects to represent real-world concepts and organize code in a way that is easy to understand, maintain, and extend.
A class is defined using the ‘class’ keyword, followed by the name of the class, and a colon. The class body is indented and contains the data and behaviour of the class.
For example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(“Hello, my name is ” + self.name)
In this example, the ‘Person’ class has two data attributes ‘name’ and ‘age’ and one behaviour ‘say_hello’ that prints a message with the name of the person.
To create an object of a class, you use the class name followed by parentheses. This is known as the instantiation
person1 = Person(“John”, 30)
In this example, an object of the ‘Person’ class is created and assigned to the variable ‘person1’. The object is initialized with the name “John” and the age 30.
You can access the data of an object using the dot notation, for example:
print(person1.name) # Output: John
You can also call the methods of an object using the dot notation, for example:
person1.say_hello() # Output: Hello, my name is John
In Python, classes can also inherit from other classes. This allows you to create a new class that inherits the data and behaviour of an existing class and can also add new data and behaviour of its own. This is known as inheritance. The class that inherits from another class is called the child class and the class that is inherited from is called the parent class.It’s important to note that python is not a pure OOP language and it allows you to use different paradigms of programming like functional programming and procedural programming.
A library is a collection of pre-written code that you can use to perform various tasks. Libraries provide a way to add functionality to your program without having to write the code yourself.
There are many libraries available for Python, some of the most popular ones include:
To use a library in Python, you first need to install it, then you can import it into your program and start using its functions and classes. You can install a library using pip, which is the package installer for Python. Once installed, you can import the library and start using it.
For example, to use the NumPy library in your program, you would first need to install it by running ‘pip install numpy’ in your command line. Then, in your Python program, you would import it using the following line of code:
import numpy
You can also use an alias name when importing a library which makes it easier to call its functions and classes by using the alias name instead of the full library name.
import numpy as np
Now you can use the library’s functions and classes by calling them with the np prefix.
It’s important to note that some libraries may have multiple versions and you should use the version that is compatible with your Python version or other libraries that you are using.
Want to Learn Python?
Learn Python with Purple Tutor by solving quizzes and interesting games. Enroll in our demo course for FREE!
Frequently Asked Questions (FAQs)
1. Can I try a free class?
A: Yes. the first demo class is free of charge. You can book the free class from the booking link.
2. What are the courses that Purple Tutor offers?
A: Purple Tutor provides Cutting edge courses to make your child future ready. We have courses like – Python, Web Development, Artificial Intelligence, Machine Learning, Cyber Security, & Roblox Games.
3. Is the coding course schedule flexible?
A: The courses for kids are flexible. You can select any time and any day that works around your child’s schedule.
4. How do I know what coding course is right for my kid?
A: The teachers assess the level of the student in the demo class on the basis of which the course is suggested.
5.Will my child receive a certificate?
A: Students get certificated after completion of each course. The certificate recognises the skills the student learnt and the level of mastery achieved.
6. What do you require to learn coding from Purple Tutor?
A: You need a laptop/computer with a webcam and a stable internet connection.
7. What level will my kid reach in coding expertise after completing your course?
A: Kids learn everything about coding that exists in their courses. They will learn basic programming concepts, algorithms, sequencing, writing code to solve puzzles, projects, geometric patterns, etc. According to the course undertaken, kids will learn as per the curriculum.