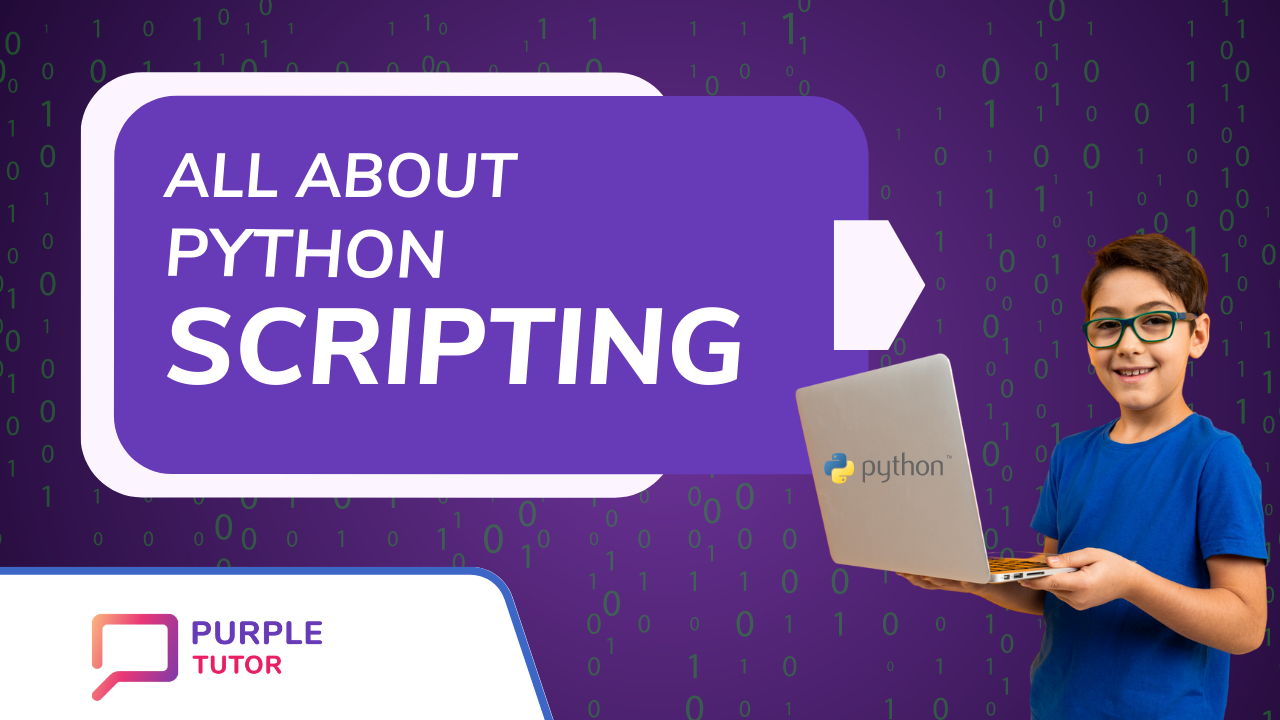
Python scripting refers to the process of using the Python programming language to write scripts or programs that automate tasks, manipulate data, or perform other operations on a computer. It is a popular scripting language because it is easy to learn, has a simple syntax, and has a wide range of libraries and tools available for it.
Python scripts are used for a variety of purposes, such as – web scraping, data analysis, automation, system administration, etc. It can be run from a command line interface or integrated into other programs or applications. The scripts can be written and executed on any operating system that supports Python, including Windows, macOS, and Linux.
Basic knowledge of Python scripts
Here’s some basic knowledge about Python scripts:
1. Python Scripts
- Python scripts are plain text files containing Python code with the .py extension.
- They are executed by the Python interpreter, which reads the code and executes it line by line.
- Python scripts are created and edited using any text editor or integrated development environment (IDE).
2. Variables and Data Types
- Variables are used to store data in Python. They can hold different types of values such as numbers, strings, lists, etc.
- Common data types in Python include integers, floats, strings, lists, tuples, dictionaries, and booleans.
- Variables are created by assigning a value to a name.
For example:
name = “Alice”
age = 25
3. Control Flow
- Python provides control flow statements like if-else, for loops, while loops, and more to control the execution flow of a program.
- if statements are used to perform different actions based on conditions.
- for loops are used to iterate over a sequence of elements.
- while loops are used to repeat a block of code until a certain condition is met.
4. Functions
- Functions are reusable blocks of code that perform a specific task.
- Functions can take parameters (inputs) and return values (outputs).
- They help in organizing code and making it more modular.
- A function is defined using the def keyword.
For example:
def greet(name):
print(“Hello”, name, “!”)
5. Input and Output
- Python provides functions to interact with the user through input and output.
- The print function is used to display output to the console.
- The input() function is used to accept user input from the console.
6. File Operations
- Python provides various functions and methods to work with files.
- You can open, read, write, and manipulate files using built-in functions and methods.
- The open() function is used to open a file, and the read(), write(), and close() methods are used for file operations.
These are some of the basic concepts in Python scripting. As you progress, you can explore more advanced topics such as modules, classes, error handling, and external libraries to expand your knowledge and capabilities in Python.
Python Scripting Examples
Here are some simple Python scripting examples:
1. Simple Calculation
a = 5
b = 3
c = a + b
print(c)
2. String Manipulation
message = “Hello, World!”
print(message.upper())
print(message.lower())
print(len(message))
2. Looping and Conditionals
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0:
print(number, “is even”)
else:
print(number, “is odd”)
3. Creating Functions
def greet(name):
print(“Hello,”, name, “!”)
greet(“Alice”)
greet(“Bob”)
4. Working with Lists
fruits = [“apple”, “banana”, “cherry”]
print(fruits[0])
print(fruits[-1])
fruits.append(“orange”)
print(fruits)
Benefits of Python Scripting
Python scripting offers several benefits that contribute to its popularity among developers. Here are some key benefits of Python scripting:
- Readability and Simplicity: Python has a clean and easy-to-read syntax, which makes it highly readable and understandable. It emphasizes code readability and minimizes the use of complex symbols or constructs, making it easier for developers to write and maintain code.
- Easy to Learn: Python has a gentle learning curve, making it accessible to beginners. Its simplicity and readability make it an ideal language for those new to programming or transitioning from other languages.
- Cross-platform Compatibility: Python is a cross-platform language, meaning it runs on various operating systems such as Windows, macOS, Linux, and more. This allows developers to write code that can be executed on different platforms without major modifications.
- Rich Ecosystem and Libraries: Python has a vast collection of libraries and frameworks that extend its capabilities. These libraries provide ready-to-use functions and modules for various tasks, such as data analysis (NumPy, pandas), web development (Django, Flask), scientific computing (SciPy), machine learning (TensorFlow, scikit-learn), and more. The availability of these libraries saves time and effort in implementing complex functionalities from scratch.
- Rapid Development: Python’s simplicity and availability of libraries enable faster development cycles. It allows developers to quickly prototype and build applications, reducing development time and increasing productivity.
- Integration and Extensibility: Python can easily integrate with other languages such as C, C++, and Java. It provides interfaces to interact with code written in other languages, enabling developers to leverage existing code and take advantage of high-performance libraries.
- Large Community and Support: Python has a vibrant and active community of developers. This means that there are ample resources, documentation, tutorials, and online communities available to seek help, share knowledge, and collaborate with other Python developers.
- Versatility: Python is a versatile language that can be used for a wide range of applications. It is suitable for web development, scientific computing, data analysis, artificial intelligence, automation, scripting, and more. Its versatility makes it a popular choice for different domains and use cases.
These benefits make Python scripting an attractive choice for both beginners and experienced developers, enabling them to build applications efficiently, quickly, and with maintainability in mind.
Frequently Asked Questions (FAQs)
1. Can I try a free class?
A: Yes. the first demo class is free of charge. You can book the free class from the booking link.
2. Is the coding course schedule flexible?
A: The courses for kids are flexible. You can select any time and any day that works around your child’s schedule.
3. How do I know what coding course is right for my kid?
A: The teachers assess the level of the student in the demo class based on which the course is suggested.
4. Will my child receive a certificate?
A: Students get certificated after completion of each course. The certificate recognises the skills the student learnt and the level of mastery achieved.
5. What do you require to learn coding from Purple Tutor?
A: You need a laptop/computer with a webcam and a stable internet connection.